Subtitles section Play video
Hey, what's up everybody?
I'd like to welcome you to another Audio Programmer tutorial.
And in this tutorial, we're going to talk about the Juice Audio Processor Editor class.
This is the base class that is used by Juice for any visual elements that you would like to use for your user interface.
That is, what people see when they open up your plugin.
The main objectives for this tutorial are to introduce you to the Audio Processor Editor class and the concept of components within Juice.
To introduce you to the concept of parent and child components.
I'll also show you how to create new source files within the CMake system to create child components.
And finally, we'll dig a little bit deeper into what happens when you load a plugin and how the editor is actually created in the plugin loading process.
As always, I'll also briefly discuss some of the core C++ concepts along the way for those who are just getting started.
If you're ready to take that deeper dive into audio plugin development, I invite you to check out our books.
The Complete Beginner's Guide to Audio Plugin Development and Creating Synthesizer Plugins with C++ and Juice.
These are great resources to get started.
All in all, there's about 900 pages on how to start creating your own plugins.
I invite you to check them out on theaudioprogrammer.com forward slash books.
Link is in the description below.
This video is also brought to you in part by our sponsor JetBrains.
If you're looking for ways to streamline your development process, I invite you to check out their amazing C-Line IDE.
Their tools have helped me to exponentially speed up my workflow and I'm proud to be a supporter.
Be sure to check out more on the link in the description below.
And with that being said, let's get started.
OK, so right now we're in a blank Juice plugin template project.
If you're not sure how to get to this point, be sure to check out our tutorial called CMake vs.
ProJuice or FaceOff, which shows you how to start creating a project that will help you get up and running.
And as I was saying before, we're going to use CMake for tutorials from here on out.
It's the industry standard.
And I think that even though it's more difficult to get started, it will definitely help you in the long run.
So where we are now is in the Audio Plugin Audio Processor Editor class.
So as we can see, we have four files that are over here on the left hand side.
Plugin Editor.cppnh and Plugin Processor.cppnh.
Plugin Processor is what we went through last tutorial where we talked about the Audio Processor class.
And that is where the actual audio processing happens for your plugins.
So not the part that you see, but the actual audio processing.
The Audio Processor Editor class is what you actually see when you load your plugin.
So if I just go ahead and I compile this here, we can see that this plugin is loaded.
We're in standalone mode right now.
And that what we actually see, this is the Audio Processor Editor.
Now, if we dive a little bit deeper into this class, we can see that it inherits from this class called the Audio Processor Editor class.
So what I'm going to do is I'm going to jump into this class now.
And what we'll see is that the Audio Processor Editor class is a juice class.
And this in turn inherits from the component class.
So the component class is essentially any visual element that you have within juice.
So when you load up some type of visual class in juice or you're creating some type of visual element, it will inherit from this component class.
And what I'll do here is I'll actually show you the juice documentation because sometimes it's nice to see it this way as well, which is that we have this Audio Processor Editor class.
And then this is what's called an inheritance diagram, which shows you where in the hierarchy these classes inherit from.
So it says here that the juice Audio Processor Editor class inherits from the juice component class.
So anything that you can do with a component class, you can do with an Audio Processor Editor class because Audio Processor Editor inherits from component.
OK, so now let's just jump back into the source code here.
Here we have four functions that are in this class.
And this is pretty typical for what you would see within any type of component or visual element class.
You would have a constructor and a destructor that are called when the window is created or destroyed.
And you have this paint function, which is more for things that you would do within that component itself.
So if you wanted to write some text, for example, we have the window currently saying hello world.
If we wanted to change the color of the background, if we wanted to change the size of the text, we would do that within paint.
And then we have this resized function, which is where we would actually lay out any type of other components that we want to sit inside of this Audio Processor Editor.
So I'm going to describe that in a little bit more detail here by going to the CPP file, which is the actual implementation of these function calls.
So as I said before, first thing that we have is the constructor.
And I won't go really into what these are because I don't think that they're relevant so much for getting started.
But what we see down here is we have this set size, which at the moment is 400 by 300 pixels.
Right.
So if we just go ahead and build that now, we see that this is 400 by 300 pixels.
And if I wanted to change this, let's say I wanted to change it to a 600 pixel height and then we just rebuild this here, then we will see that now the plugin has got taller.
Right.
So as I was saying before, for people who are just getting started, the constructor is what's being called when the window is first created.
And then the destructor is what happens when the when the window is destroyed.
Now, one thing that I will say that was a common mistake that I used to think about when I was first getting started and one that I see very often for other people who are getting started is that when when we're in a DAW, let's say we're in Ableton Live or in Reaper, that it's very easy to think that if we close out the plugin, that the plugin window is actually being minimized.
That's how I used to think of it in my mind, because when you pull up the window again, all of the parameters and dials and sliders are all in the same place where you left them.
Or at least they're supposed to be if the person made the plugin properly.
So because of that, I used to think that this window was actually being minimized.
But one thing one key thing to think about is that the window is actually being destroyed.
So it actually does not exist anymore.
You'll learn a little bit more about this as we get a little bit further in the plugin development.
But one great example of where this mistake is made is when it comes to sliders that are holding some type of value.
So when it's holding that value, it's very important that the value of the slider that is sitting within this plugin window is able to relay what the value of the slider is over to the plugin processor.
Because if it doesn't, and you're relying on that value to be there, and the plugin window is destroyed, then it doesn't exist anymore.
And you're going to be trying to look for a value that does not exist.
So once again, that gets a little bit, that's jumping ahead a little bit.
But it's just something to keep in mind that as you're going through this journey, that when you're creating this window, that when you close it out, it's not minimized.
It actually does not exist anymore.
Okay.
So jumping down a little bit further, we have this function that's called paint.
So paint, I would think of as what you actually want to happen within this visual component itself.
So as we can see, we have this g.fillall, which is filling the background of the component with this green color.
Now, what I could do is I could actually change this color.
Let's just go ahead and change it to black.
And what I can do is, oh, I need to get rid of this bracket.
And what I could do is I could recompile this.
And then what we should see is now the plugin window is black, right?
And if I wanted to change this text to red, and I wanted to make it a little bit bigger, I would just do that here.
So the size of this text is being set by this g.setfont.
So if I wanted to change this from 15 to 30, and then if I wanted to change the color from white to red, this is where all of that would happen.
So paint, once again, is the place where the things that are actually happening within this component itself are being changed.
Now coming down to the resize function, we see that there's nothing that's happening inside of this at the moment.
And this brings us to another principle of these component classes, which is that components can hold other components.
So when I say component, think a window, like a visual window.
And these components can have other components inside of them.
And the way that you would lay those out is typically in this resized function.
Okay, so what we're going to do now is we're going to create our own component class.
And we're going to include it as a child within this plugin editor class that we have here.
Okay, so if that sounds like a daunting task, don't worry, I'm going to take you step by step.
And I feel confident that if you follow along, that you're going to come out successfully on the other side.
So let's go ahead and get started.
First thing that we're going to need to do if we want to create a component class is we're going to need to create some source files.
So the way that we're going to do that is we're going to go over here to our source folder over here in our Explorer.
We're going to right click and create a new C++ class.
So this is how you would do it in SceneLion.
If you were working in Xcode or Visual Studio, it essentially be a similar type of command.
So now I'm going to go ahead and create this.
And I will just call this, let's just call it square.
And I will create a .cpp and .header file for this new class that we're calling square.
And I'm going to hit OK.
And now we've created those new source files.
I'm just going to hit add.
And now we have some new source files.
And what I'm going to do is I'm just going to get rid of everything just so it's blank.
And we'll start from complete scratch.
The next thing that we need to do is we need to now include this within our CMake file.
So the reason that we need to do this is if we look at our current CMake file, we can see here that the source files that we've included so far are plug-in editor.cppnh and plug-in processor.cppnh.
And so it doesn't know at the moment that square.cppnh are supposed to be part of our build system, part of the files that we want to use and include in our project.
So just to show you here, if I go into the source folder and just open it up in the finder, here we are in our project.
You see cmakelist.txt here.
And there's the source folder.
I'm just going to double-click in there.
And there we see our class, all of our source files, in fact, square.cppnh and all of our other ones.
Now, what we need to do is we need to include this in our CMake file.
Now, the reason that you need to say source slash dot plug-in editor and slash square dot h and cpp is because the declaration that you're making inside the CMake file needs to be relative to where the cmakelist.txt resides.
So here we are in our project.
We see that we have this cmakelist.txt, and then we have this source folder.
So we need to say relative to the cmakelist.txt file, we're going into source and into these files.
If we had another folder that we just, let's say we called it code, for instance, and we put these files in here, then in our cmakelist.txt, it would need to say source slash code slash whatever your source file is.
So that's the reason why the convention is being named this way.
Okay, so now we're going to put our square class files in here.
So we've got square.cpp, source, square.h.
And now those are included.
Now, if you're in Visual Studio or in Xcode, what you're going to need to do to update your CMake build is you're going to need to invoke CMake, which is that normal command that you normally do, which is cmake-bbuilds-gxcode or whatever your command is, right?
If you're here in SceneLine, it actually has CMake integrated into the IDE, and I can just hit this little reload CMake project, and it'll actually run CMake here inside the IDE.
So that's one of the things I really love about CMake here in SceneLine.
So there we go.
So that's run, and now we're all good to go.
So now we have this square.h source file, and we're going to build this from scratch.
So the first thing that we're going to do is we're going to declare pragma once.
The reason that we do this is because what we need to do is we're going to need to include some other source files to be able to give this class that we're creating some functionality.
So right now this source file knows nothing about Guice.
It knows nothing about anything except for basic C++, and what we're going to need to do is include a graphics library from Guice in order to be able to inherit the component class, which allows us to create the window, okay?
If we had another class that was using another include that was using the graphics library, and we included it, what pragma once does is it creates us from having name collisions where you're including source files more than one time.
So it says, okay, he's already included the Guice graphics library, so we don't need to include that again.
So that's what pragma once is for.
So the first thing that we'll do is we'll just create this basic class.
The way we do this is called class.
I'm just going to call this square, and now I've created my class, okay?
Now square does not have any functionality right now, so it doesn't know anything about being a Guice component, doesn't know anything about Guice, doesn't know anything about anything except for basic C++.
In order for us to be able to give square the functionality that it needs, we need to be able to inherit from the Guice component class, okay?
So the way that we do that is that if we look to our Guice documentation, you'll see that we want to inherit this component class to give it the functionality where it can draw a window within our project.
Now, Guice is a collection of libraries.
So you have DSP libraries, you have graphics libraries, you have all of these different libraries that you can use.
The way that you can find out what library that you need to include is here in the Guice documentation.
If you look below this Guice component class reference, it'll normally have the name of the library that you need to include right here, which in this case is Guice underscore GUI underscore basics.
So the way that you would do this is here we go below the pragma once.
And what I will do is I'll do a hashtag include.
And I'm actually going to get rid of the quotes because one thing that I learned is that for library includes, you actually use angle brackets rather than quotes for those.
So now what I can do is I can use Guice GUI basics.
And so that leads us to the folder that it's in.
And then Guice GUI basics dot H.
OK, so now what we can do is we can we can now invoke anything that's within this Guice GUI basics library.
OK, there's another way that you can that you can do this.
That's where you can say include Guice header dot H and that will give you access to all of the Guice library code.
But I would say that this is the more correct and more optimized way to do this.
OK, so now what we can do is we can inherit Guice component.
So the way that we do this is we just put a little colon here and we need to declare it as public or private first.
So we want this to be a public class.
And then we got Guice colon colon component.
OK, so now we've inherited the Guice component class.
So now what we can do is we can call upon in this class anything that that a Guice component is able to do.
OK, so a lot of the next functionality that we're going to need is going to be very similar to what we've done with the plugin editor.
OK, so here in the plugin editor we have four functions which are the constructor, the destructor, paint and resized.
To keep things super simple, I'm going to just do paint and resize.
And what I'll do is I'll just literally copy here from this audio processor editor.
And I'm just going to paste it in square.h.
The reason that I'm not worrying about including the constructor or the destructor is because we don't need to do anything with that in this tutorial.
So I'm not going to complicate it for you.
So now what I'm going to do is I'm going to go here into this class square.
And these need to be public functions that we can publicly access.
And I'm just going to go ahead and paste those in like so.
OK, because we want very similar functionality to what we have with our with our plugin editor class, which is also a Guice component.
So we're going to want to just to review going back to plugineditor.cpp.
In this in this situation, we're going to want to just give our component some color.
So fill the the rectangle with a color and maybe do some writing.
And that's it.
In fact, we probably don't need resized for to put in this square class, but I'll just do it just for just just to show you how to do it.
So the next thing that we need to do is we need.
So now we've declared these functions.
What we need to do is we need to provide implementations for these functions.
So the way that we do this is go over to square.cpp.
Make sure that you have hashtag include square.h there or else it won't know what you're talking about.
And what I'm going to do is I'm just going to paste.
I'm just going to paste these in there.
And just to go back to square.h for for a second, just to reiterate, we are doing the override keyword for for paint and for resize because those are functions that are created from Guice component.
So we have if we look at paint.
We can see that that's a virtual function.
So the reason that we wanted that we want to include this is because we want to be able to to paint our component a certain color.
And in order to do that, we need to include the paint functionality, which is happening through this function.
So that's the reason why this is happening.
So now to implement these functions, I'm going to just put some angle brackets here.
Curly brackets, as they call them.
Now, there's one more thing that we need to do, and this is just something I'm once again reiterating for people who are just getting started, which is that.
If you have a lot of paint functions, if you have a lot of components, then then when your code is compiling, it doesn't know which paint you're referring to when when you're trying to call this particular paint.
OK, because we don't have what's called a qualified class name in front of it.
So because we want to call the paint function that's specific to square, what we want to do is we want to do this.
Which is we're saying that we want to call these the paint function, but the paint function that is specific to the class square.
OK, so that's once again, just kind of basics of C++, but but stuff that stuff that is essential to know.
And so I did that for resized as well.
Now from here, now we have a component class.
OK, now we just need to give it a little bit of functionality.
And the way that we'll do this, once again, I'll just go over here to the plugin editor that CPP.
And, you know, if you don't if you don't have these these calls memorized, you know, all you need to do is just copy it from something that works and just pop it in there and change a few things.
OK, so that's a great way to get started.
So first thing that we see is that we are getting an error here on this G.
It doesn't know what G is, and that's because it just says juice graphics ampersand and it doesn't have a doesn't have a variable name there.
So I just need to pop that G in there for it to know what what the graphics object is or just to be able to refer to that.
Now, what I'm going to do is I can just change a few things around.
OK, so if I'm going to change the background color, I can change it here.
Let's just change it to orange.
And then we'll set the color of the of the font to white.
I'm just going to make it a little bit smaller.
And then.
I will just.
Say here the audio programmer.
So there we go.
So I've just changed a couple of things around, repurpose some code that I found in a component class that already works.
And so now we have a component class that we've just created.
So congratulations, you have your very first component class.
So now if we build, we just want to make sure that this code is working OK so far.
And we can see that we don't see anything else.
We don't see this window that we've just created.
The reason that we don't see it is because we haven't actually included it as a child to this to to our main window.
So the way that we need to do this is we need to go back and we need to go into our plug in our plug in editor class.
And now what we need to do is we need to include square dot H.
OK, because that's that's the component that we've just created.
So here I'm going to do a include.
Square dot H.
So now I have said that I want to bring in this code from square H and be able to do something with it.
And now what I want to do.
Is I want to create a square object.
I want to create a square component.
I'm just going to call this square.
And now I've created an object within our editor.
And now what I'm going to do is go to the CPP file.
I'm going to go up here to the constructor and they have this special function that is specific to the component class called add and make visible.
Now what this does, if we go back to our documentation.
And if I can get to it quickly.
So it says adds a child component to this one and makes the child visible if it isn't already.
OK, so so that's what that's doing.
So remember that concept earlier that I was explaining about parent and child components.
What we're doing is we're making square a child component of audio plug in audio processor editor.
So we're saying we want we want square to be a window that's inside this other window that we've that was already created for us.
Our main plug in window.
The last thing that we need to do is we need to actually put it on the screen and say where we want it to be on the screen.
And we do that in this resized function.
And the way that we do that is we would say square.
And then there are many different ways to do this.
Many, many different ways to do this.
The easiest is using this set bounds function here.
And so if we go back to the documentation again.
If we go to set bounds.
We'll see we'll see that there are a lot of different ways that you can that you can actually set bounds.
The way that we're going to use in this instance is just using an X a Y giving it a width and giving it a height in pixels.
So we're just saying where we where we want to set it on the X axis.
Set it where we want to set it on the Y axis and how wide and how tall we want it to be.
Just a note that component classes are rectangles and that the X and Y position will be relative to the top left of the rectangle.
OK.
So not to the center but to the top left.
So just a little thing to keep in mind there.
So we're going to give it an X position.
Just make it 100. 100.
And then I'll give it a width.
Let's say that it's 200 by 200.
And now we're going to go ahead and build it.
And if everything works properly we should see a square within another square.
There we go.
So now we have created a child component that's within this larger component here.
And as you can see it says the audio programmer inside of it.
The background is orange.
Now if I wanted to change the width and the height of this I could do this very easily just by just by doing this and just refreshing again.
And here we go.
And now it's smaller.
So that's essentially how you start how you get started with the juice component class and including that in the juice audio processor editor.
Now the final thing that I'll show you is diving a little bit deeper into how the plug-in actually gets created.
So for people who want to really understand how does this process actually get started.
What we can do is we can go back to our plug-in processor dot CPP.
And there's actually a function in here called create editor.
And what you're going to see is that this is that it creates a new audio plug-in audio processor editor which is what the name of this class is.
Right.
So that's that's where that's where this actually gets created.
So if you look at the juice plug-in process in terms of how that actually what actually starts creating where where things start and what things get created and in what order.
You'll see here that the audio processor gets created first and then it creates a new audio processor editor.
And that's and that's what that's what you see here.
So if you didn't want it to have a plug-in window you could just you could just return it false.
But that just shows you that it's the audio processor that starts.
So it's the background audio processing that starts first and then it creates the visual aspect and does the connection points of that.
So I hope that this was educational for you and that that you found it helpful.
One thing that I should mention is that this is one of a number of ways that user interfaces can now be created using juice.
I think that this is the easiest way to get started.
But once you get a little bit more advanced there are actually ways that you can create user interfaces using JavaScript as well.
But that's a little bit more complex to set up and get running.
But we'll go through that a little bit later.
So I hope you enjoy this.
If you liked it, please give it a like and subscribe to our channel and I will see you next time.
Happy coding.
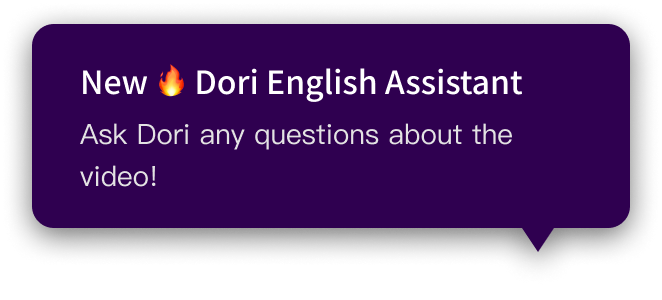